※ Reporting bugs or issues will help me improve the plugin and help others.
CustomTabUI
● This .cs for new style tab UI implementation for developer.
● Supports FullLine Tab, HalfLine (Left/Right) Tab (v0.2)
● Supports tab hierarchy traversing function (v0.3)
● Supports dynamic tab append/delete function (v0.3)
● Supports auto hide for UI elements when changing tabs. (no need to remove UI elements manualy)
● Supports tabs using icons as well as text
● For verification of CustomTabUI, I used the library in AutoFlutterHip in the link below.
hub.virtamate.com
● You can extract CustomTabUI.cs and free modify/use it. CustomTabUI.cs does not have any dependencies
The code below is all you need.
Also, The code below is all you need.
The code below is all you need, too.
The code below is all you need.
Credits :
MacGruber Utils / By: MacGruber / License: CC-BY
hub.virtamate.com
I extracted some of the functionality for creating the UI elements needed for Example1,2,3,4 from [MacGruber_Utils.cs] and placed it into [Utils.cs].
As always, Thanks to @MacGruber for sharing his beautiful code !!!
CustomTabUI
● This .cs for new style tab UI implementation for developer.
● Supports FullLine Tab, HalfLine (Left/Right) Tab (v0.2)
● Supports tab hierarchy traversing function (v0.3)
● Supports dynamic tab append/delete function (v0.3)
● Supports auto hide for UI elements when changing tabs. (no need to remove UI elements manualy)
● Supports tabs using icons as well as text
● For verification of CustomTabUI, I used the library in AutoFlutterHip in the link below.
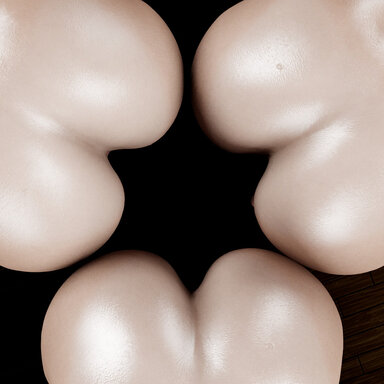
AutoFlutterHip - Plugins + Scripts -
※ Reporting bugs or issues will help me improve the plugin and help others. This plugin consists of two parts: AutoFlutterHip and AutoFlutterHipTechnic. AutoFlutterHip ● This plugin can apply morph depending on the angle at which you bend your...

● add : support dynamic tab append/delete function
● add : support tab hierarchy traversing
● fix : failed to process (JSONStorableAction)
● fix : minor bug fix
● add : support tab hierarchy traversing
● fix : failed to process (JSONStorableAction)
● fix : minor bug fix
C#:
CustomTabUI tui = new CustomTabUI(this, CreateUIElement);
Tab a = tui.newTab(this, "TabA");
{
a.add(initSlider("SliderA"));
a.add(initToggle("ToggleA"));
}
Tab b = tui.newTab(this, "TabB");
{
b.add(initSlider("SliderB"));
b.add(initToggle("ToggleB"));
}
---- full traverse ----
foreach (KeyValuePair<String, Tab>pair in tui.getTabs())
{
String key = pair.Key; // TabA, TabB key
Tab tab = pair.Value; // TabA, TabB instance
for (int i = 0; i < tab.elements.Count; i++)
{
object o = elements[i]; // JSONStorableParam or JSONStorableAction
}
}
---- key traverse ----
Tab a = tui.getTab("TabA");
Tab b = tui.getTab("TabB");
JSONStorableFloat j == a.get("SliderA") == tui.getElement("TabA", "SliderA") as JSONStorableFloat;
JSONStorableBool j == a.get("ToggleA") == tui.getElement("TabA", "ToggleA") as JSONStorableBool;
JSONStorableFloat j == b.get("SliderB") == tui.getElement("TabB", "SliderB") as JSONStorableFloat;
JSONStorableBool j == b.get("ToggleB") == tui.getElement("TabB", "ToggleB") as JSONStorableBool;
---- also, dynamic obtaining UIDynamic via JSONStorable ----
UIDynamicSlider u = Tools.JSONStorable2UIDynamic(instance of JSONStorableFloat) as UIDynamicSlide;
UIDynamicToggle u = Tools.JSONStorable2UIDynamic(instance of JSONStorableBool) as UIDynamicToggle;
UIDynamicColorPicker u = Tools.JSONStorable2UIDynamic(instance of JSONStorableColor) as UIDynamicToggle;
UIDynamicTextField u = Tools.JSONStorable2UIDynamic(instance of JSONStorableString) as UIDynamicTextField;
UIDynamicPopup u = Tools.JSONStorable2UIDynamic(instance of JSONStorableStringChooser) as UIDynamicPopup;
UIDynamicButton u = Tools.JSONStorable2UIDynamic(instance of JSONStorableAction) as UIDynamicButton;
● add : Support Left/Right Side Tab
● add : Support Change Tab Text Color
● add: Support Change Tab Background Color
● fix : minor bug fix
● add : Support Change Tab Text Color
● add: Support Change Tab Background Color
● fix : minor bug fix
The code below is all you need.
C#:
using TabUI;
CustomTabUI tabuiL;
CustomTabUI tabuiR;
at Init() method :::
tabuiL = new CustomTabUIHalf(this, CreateUIElement, "TabTextUI-L", true, selctedTab)
.setTextColor(new Color(1f, 1f, 1f, 0.3f), Color.yellow)
.setBackgroundColor(new Color(0.53f, 0.19f, 0.32f, 0.9f));
for (int i = 0; i < 4; i++)
{
String txt = "Tab " + i.ToString();
Tab tab = tabuiL.newTab(this, txt, 80);
tab.add(Utils.SetupButton(this, txt + " - button (left)", null, true));
tab.add(Utils.SetupToggle(this, txt + " - toggle (left)", false, true));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (left)", 0f, 0f, 1f, true));
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (left)", new List<string>{ "None", "1", "2", "3" }, true));
tab.add(Utils.SetupColor(this, txt + " - color picker (left)", Color.white, true));
tab.add(Utils.SetupSpacer(this, 10, true));
}
////
Icon icon = new Icon(this, 20, 4, 36, 36, "/src/resource/star(64x64)_t_w.png");
tabuiR = new CustomTabUIHalf(this, CreateUIElement, "TabTextUI-R", false, selctedTab);
for (int i = 0; i < 4; i++)
{
String txt = "Tab " + i.ToString();
Tab tab = tabuiR.newTab(this, txt, 80, icon);
tab.add(Utils.SetupColor(this, txt + " - color picker (right)", Color.white, false));
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (right)", new List<string>{ "None", "1", "2", "3" }, false));
tab.add(Utils.SetupButton(this, txt + " - button (right)", null, false));
tab.add(Utils.SetupToggle(this, txt + " - toggle (right)", false, false));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (right)", 0f, 0f, 1f, false));
tab.add(Utils.SetupSpacer(this, 10, false));
}
at Start() method :::
if (tabuiL != null) tabuiL.selectTab(0); // select first tab
if (tabuiR != null) tabuiR.selectTab(0); // select first tab
at OnDestroy() method :::
if (tabuiL != null) tabuiL.close(this);
if (tabuiR != null) tabuiR.close(this);
Also, The code below is all you need.
C#:
using TabUI;
CustomTabUI tabui;
at Init() method :::
tabui = new CustomTabUI(this, CreateUIElement, "TabTextUI")
.setTextColor(new Color(1f, 1f, 1f, 0.3f), Color.yellow)
.setBackgroundColor(new Color(0.53f, 0.19f, 0.32f, 0.9f));
for (int i = 0; i < 8; i++)
{
String txt = "Tab " + i.ToString();
Tab tab = tabui.newTab(this, txt);
tab.add(Utils.SetupButton(this, txt + " - button (left)", null, true));
tab.add(Utils.SetupToggle(this, txt + " - toggle (left)", false, true));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (left)", 0f, 0f, 1f, true));
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (left)", new List<string>{ "None", "1", "2", "3" }, true));
tab.add(Utils.SetupColor(this, txt + " - color picker (left)", Color.white, true));
tab.add(Utils.SetupSpacer(this, 10, true));
tab.add(Utils.SetupButton(this, txt + " - button (right)", null, false));
tab.add(Utils.SetupToggle(this, txt + " - toggle (right)", false, false));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (right)", 0f, 0f, 1f, false));
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (right)", new List<string>{ "None", "1", "2", "3" }, false));
tab.add(Utils.SetupColor(this, txt + " - color picker (right)", Color.white, false));
tab.add(Utils.SetupSpacer(this, 10, false));
}
at Start() method :::
if (tabui != null) tabui.selectTab(0);
at OnDestroy() method :::
if (tabui != null) tabui.close(this);
The code below is all you need, too.
C#:
using TabUI;
CustomTabUI tabui;
at Init() method :::
Icon ico0 = new Icon(this, 20, 4, 36, 36, "/src/resource/woman2(64x64)_t_w.png");
Icon ico1 = new Icon(this, 20, 4, 36, 36, "/src/resource/businessperson(64x64)_t_w.png");
Icon ico2 = new Icon(this, 20, 4, 36, 36, "/src/resource/star(64x64)_t_w.png");
Icon ico3 = new Icon(this, 20, 4, 36, 36, "/src/resource/flower(64x64)_t_w.png");
Icon[]icon = new Icon[] { ico0, ico1, ico2, ico3 };
tabui = new CustomTabUI(this, CreateUIElement, "TabTextUI", selctedTab);
for (int i = 0; i < 8; i++)
{
String txt = "Tab " + i.ToString();
Tab tab = tabui.newTab(this, txt, 80, icon[i%4]);
tab.add(Utils.SetupButton(this, txt + " - button (left)", null, true));
tab.add(Utils.SetupToggle(this, txt + " - toggle (left)", false, true));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (left)", 0f, 0f, 1f, true));
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (left)", new List<string>{ "None", "1", "2", "3" }, true));
tab.add(Utils.SetupColor(this, txt + " - color picker (left)", Color.white, true));
tab.add(Utils.SetupSpacer(this, 10, true));
tab.add(Utils.SetupButton(this, txt + " - button (right)", null, false));
tab.add(Utils.SetupToggle(this, txt + " - toggle (right)", false, false));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (right)", 0f, 0f, 1f, false));
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (right)", new List<string>{ "None", "1", "2", "3" }, false));
tab.add(Utils.SetupColor(this, txt + " - color picker (right)", Color.white, false));
tab.add(Utils.SetupSpacer(this, 10, false));
}
at Start() method :::
if (tabui != null) tabui.selectTab(0);
at OnDestroy() method :::
if (tabui != null) tabui.close(this);
C#:
using TabUI;
CustomTabUI TabTextUI = null;
CustomTabUI TabIconUI = null;
CustomTabUI TabOther1 = null;
CustomTabUI TabOther2 = null;
at Init() method :::
TabTextUI = initTabTextUI();
TabIconUI = initTabIconUI();
TabOther1 = initTabOther1();
TabOther2 = initTabOther2();
CustomTabUI initTabTextUI()
{
String[]label = new String []{ "This is a Tab Zero", "Tab one", "Customazing Tab two", "T03" };
int []width = new int []{ 300, 140, 340, 80 };
CustomTabUI tabui = new CustomTabUI(this, CreateUIElement, "TabTextUI", selctedTab)
.setTextColor(new Color(1f, 1f, 1f, 0.3f), Color.yellow)
.setBackgroundColor(new Color(0.53f, 0.19f, 0.32f, 0.9f));
for (int i = 0; i < 4; i++)
{
String txt = label[i];
Tab tab = tabui.newTab(this, txt, width[i]);
tab.add(Utils.SetupButton(this, txt + " - button (left)", null, true));
tab.add(Utils.SetupButton(this, txt + " - button (right)", null, false));
if (i%2 == 1) tab.add(Utils.SetupToggle(this, txt + " - toggle (left)", false, true));
if (i%2 == 1) tab.add(Utils.SetupToggle(this, txt + " - toggle (right)", false, false));
}
return tabui;
}
CustomTabUI initTabIconUI()
{
Icon ico0 = new Icon(this, 20, 4, 36, 36, "/src/resource/woman2(64x64)_t_w.png");
Icon ico1 = new Icon(this, 20, 4, 36, 36, "/src/resource/businessperson(64x64)_t_w.png");
Icon ico2 = new Icon(this, 20, 4, 36, 36, "/src/resource/star(64x64)_t_w.png");
Icon ico3 = new Icon(this, 20, 4, 36, 36, "/src/resource/flower(64x64)_t_w.png");
Icon[]icon = new Icon[] { ico0, ico1, ico2, ico3 };
CustomTabUI tabui = new CustomTabUI(this, CreateUIElement, "TabTextUI", selctedTab);
for (int i = 0; i < 8; i++)
{
String txt = "Tab " + i.ToString();
Tab tab = tabui.newTab(this, txt, 80, icon[i%4]);
tab.add(Utils.SetupButton(this, txt + " - button (left)", null, true));
tab.add(Utils.SetupButton(this, txt + " - button (right)", null, false));
tab.add(Utils.SetupToggle(this, txt + " - toggle (left)", false, true));
tab.add(Utils.SetupToggle(this, txt + " - toggle (right)", false, false));
if (i%2 == 1) tab.add(Utils.SetupSliderFloat(this, txt + " - slider (left)", 0f, 0f, 1f, true));
if (i%2 == 1) tab.add(Utils.SetupSliderFloat(this, txt + " - slider (right)", 0f, 0f, 1f, false));
if (i%2 == 1) tab.add(Utils.SetupStringChooser(this, txt + " - chooser (left)", new List<string>{ "None", "1", "2", "3" }, true));
if (i%2 == 1) tab.add(Utils.SetupStringChooser(this, txt + " - chooser (right)", new List<string>{ "None", "1", "2", "3" }, false));
}
return tabui;
}
CustomTabUI initTabOther1()
{
String[]label = new String []{ "Tab0", "Tab1", "Tab2", "Tab3", "Tab4", "Tab5", "Tab6", "Tab7" };
int []width = new int []{ 100, 80, 100, 80, 100, 80, 100, 80 };
Icon icon = new Icon(this, 20, 4, 36, 36, "/src/resource/star(64x64)_t_w.png");
CustomTabUI tabui = new CustomTabUIHalf(this, CreateUIElement, "TabTextUI", true, selctedTab)
.setTextColor(new Color(0.6f, 0.2f, 0.6f, 0.9f), Color.black)
.setBackgroundColor(new Color(0.13f, 0.69f, 0.32f, 0.6f));
for (int i = 0; i < 4; i++)
{
String txt = label[i];
Tab tab = tabui.newTab(this, txt, width[i], i%2 == 0 ? null : icon);
tab.add(Utils.SetupButton(this, txt + " - button (left)", null, true));
tab.add(Utils.SetupToggle(this, txt + " - toggle (left)", false, true));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (left)", 0f, 0f, 1f, true));
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (left)", new List<string>{ "None", "1", "2", "3" }, true));
if (i%2 == 1) tab.add(Utils.SetupColor(this, txt + " - color picker (left)", Color.white, true));
}
return tabui;
}
CustomTabUI initTabOther2()
{
String[]label = new String []{ "Tab0", "Tab1", "Tab2", "Tab3", "Tab4", "Tab5", "Tab6", "Tab7" };
int []width = new int []{ 80, 100, 80, 100, 80, 100, 80, 100 };
Icon icon = new Icon(this, 20, 4, 36, 36, "/src/resource/flower(64x64)_t_w.png");
CustomTabUI tabui = new CustomTabUIHalf(this, CreateUIElement, "TabTextUI", false, selctedTab)
.setTextColor(new Color(1f, 1f, 1f, 0.3f), Color.yellow)
.setBackgroundColor(new Color(0.23f, 0.19f, 0.82f, 0.9f));
for (int i = 0; i < 4; i++)
{
String txt = label[i];
Tab tab = tabui.newTab(this, txt, width[i], i%2 == 1 ? null : icon);
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (right)", new List<string>{ "None", "1", "2", "3" }, false));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (right)", 0f, 0f, 1f, false));
tab.add(Utils.SetupToggle(this, txt + " - toggle (right)", false, false));
tab.add(Utils.SetupButton(this, txt + " - button (right)", null, false));
if (i%2 == 1) tab.add(Utils.SetupColor(this, txt + " - color picker (right)", Color.white, false));
}
return tabui;
}
at Start() method :::
if (TabTextUI != null) TabTextUI.selectTab(0);
if (TabIconUI != null) TabIconUI.selectTab(0);
if (TabOther1 != null) TabOther1.selectTab(0);
if (TabOther2 != null) TabOther2.selectTab(0);
at OnDestroy() method :::
if (TabTextUI != null) TabTextUI.close(this);
if (TabIconUI != null) TabIconUI.close(this);
if (TabOther1 != null) TabOther1.close(this);
if (TabOther2 != null) TabOther2.close(this);
The code below is all you need.
C#:
using TabUI;
CustomTabUI tabui;
at Init() method :::
Utils.SetupButton(this, "Append Tab", addTab, true);
Utils.SetupButton(this, "Delete Tab", delTab, false);
tabui = new CustomTabUI(this, CreateUIElement, "TabTextUI");
public void addTab()
{
SuperController.LogMessage("[INF] append Tab ...");
String txt = "Tab " + tabui.getTabCount().ToString();
Tab tab = tabui.newTab(this, tabui.isValid(txt) ? txt : avoidDuplecatedName(txt));
tab.add(Utils.SetupButton(this, txt + " - button (left)", null, true));
tab.add(Utils.SetupToggle(this, txt + " - toggle (left)", false, true));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (left)", 0f, 0f, 1f, true));
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (left)", new List<string>{ "None", "1", "2", "3" }, true));
tab.add(Utils.SetupColor(this, txt + " - color picker (left)", Color.white, true));
tab.add(Utils.SetupSpacer(this, 10, true));
tab.add(Utils.SetupButton(this, txt + " - button (right)", null, false));
tab.add(Utils.SetupToggle(this, txt + " - toggle (right)", false, false));
tab.add(Utils.SetupSliderFloat(this, txt + " - slider (right)", 0f, 0f, 1f, false));
tab.add(Utils.SetupStringChooser(this, txt + " - chooser (right)", new List<string>{ "None", "1", "2", "3" }, false));
tab.add(Utils.SetupColor(this, txt + " - color picker (right)", Color.white, false));
tab.add(Utils.SetupSpacer(this, 10, false));
tabui.redraw(); // repaint ...
}
public void delTab()
{
SuperController.LogMessage("[INF] delete Tab ... ");
tabui.delTab(this, tabui.getSelectSeq());
tabui.redraw(); // repaint ...
}
at OnDestroy() method :::
if (tabui != null) tabui.close(this);
MacGruber Utils / By: MacGruber / License: CC-BY
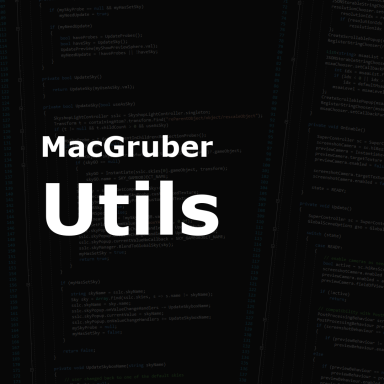
MacGruber Utils - Plugins + Scripts -
Collection of various utility functions helpful for plugin development. You should not need to manually download this, unless you are a plugin creator. This is a separate release of my MacGruber_Utils.cs which I'm using in most of my plugins. I...

As always, Thanks to @MacGruber for sharing his beautiful code !!!